Introduction
For the implementation phase of the mobile development course that I undertook during the final-year of my undergraduate degree, I was tasked with developing an application for the android operating system. In tackling this project, I focused on the informational side of Android applications in the form of a football scores and standings app that would be capable of retrieving up-to-date information on the table and fixtures of the Spanish first division, “La Liga” upon the user’s request. Aside from personal interest, this project was seen as an ideal opportunity to experiment with internet-based interactions from within the Android operating system as well as to gain experience in developing a clean and intuitive user interface that matches form and function in equal measure to provide seamless data access.
Development
Before app development could begin, the prerequisite step of locating a data source for the football information needed to be addressed. For this purpose, several football-focused API solutions were investigated so an informed decision could be made on what API was best suited for this application. This research culminated in the selection of the football-data.org API, which, as a freely accessible RESTful API, provided the perfect platform for the efficient retrieval of the data needed to form the backbone of this app. Development for the app itself started with the empty views activity template, with the first programming task being the implementation of a bottom navigation bar to cycle between the three intended fragments for this app: the upcoming games, the past results, and the league table. The decision to pursue a custom navigation bar over the navigation bar included in an alternate Android template was made for customisability reasons; the granular control provided by custom creation allowed for it to be better tailored to the app’s specific needs, resulting in a better user experience.
The following requirement was implementing a reliable method to retrieve the API data for convenient integration into the app’s various fragments, a feat that was achieved through the use of the RetroFit client. Other options, such as the more low-level OKHTTP and the native HttpUrlConnection, were also considered for this task. However, the reduction in boilerplate code afforded by a higher level of abstraction and additional features such as in-built JSON parsing made RetroFit a much more elegant solution for this task. A method then had to be devised to sensibly structure the incoming data for easy retrieval elsewhere in the application. This involved the creation of a dedicated class that would separate the JSON keys into individual subclasses, each with its own getters and setters that could then be referenced to access the specific value required wherever needed. Special consideration was also made to store the API key in the “local.properties” file to ensure that this sensitive information wasn’t leaked to the git repository.
The first screen approached during development was the display of a league table. Naturally, a table layout was the most sensible way to neatly format the data for easy readability. However, the insertion of the up-to-date data was more complex. The information for each team had to be sequentially read from the data class so new rows could be dynamically added until the entire table was formed. While another, more straightforward, approach to this feature would have been a table with a predefined number of rows corresponding to the number of teams in La Liga for simple text view manipulation, this approach lacked flexibility, stopping the easy transition between leagues that contain a different number of teams, closing a potential avenue for the future expansion of the app where the functionality would exist for multiple leagues to be viewed, hence why this solution was not opted for over the dynamic row solution. After all formatting stages were completed, a minor issue was encountered where the table was larger than the height of the screen view, so the table layout was wrapped in a scroll view as a remedy, allowing the user to navigate the whole table through simple swipes.
With the development of the league table complete, the focus shifted to the results fragment. This screen called for the dynamic generation of a number of cards, each representing a football fixture in a list format so that a user could scroll through numerous football results at a glance. The simplest solution to this was a list view, which would generate a new view for each card as it was set to become visible and destroy the view of cards leaving the screen to preserve memory. This solution would be an effective one. However, the process of perpetually generating and destroying views was needlessly resource intensive, inspiring the implementation of a recycler view that would instead retain a pool of views that could be infinitely reused, removing this overhead from the equation, and ultimately leading to a more efficient and responsive app. This functionality was managed through a separate adapter class that managed the constant binding between the API and the views to provide the seamless updates and efficient recycling that make recycler views such an advantageous solution.
Displaying the upcoming matches worked similarly to how the past results were displayed, once more using a recycler view to dynamically populate the screen with scorecards. However, this variation of the implementation was somewhat more complex due to the requirement to display data from two different API calls, as it was intended for this screen to display both the status of live football games and scheduled football games. As both types of scorecards had individual requirements, logic had to be established to differentiate the two during the binding process and allow each to have unique properties. Furthermore, a “createAdapter” function had to be placed into the fragment code to ensure that the adapter would only be created once both API calls had been successfully made to prevent a null pointer error from crashing the app.
The final feature added to this project was a dialog fragment that would appear each time a match card was pressed, fulfilling the role of providing a deeper insight into the match by providing information such as venue and half-time score. This information-rich format was designed to complement the more minimalist design of the base screen that focused on reducing visual clutter for easy readability. On a technical level, this feature involved adding an “onClick” method to each match card to manage the summoning of the dialog fragment, ensuring that the match ID was transmitted in the process to allow for a fresh API call to be made that detailed the information from that specific fixture. While this specific implementation was somewhat limited on the additional information it could provide due to limitations in the API, the formatting remains highly expandable, allowing for the easy expansion into more in-depth match information such as lineups and goal scorers should this app be progressed to the point where paid API solutions are taken into consideration.
App Walkthrough
Upon launching the app, the opening screen is the ongoing and upcoming matches screen, which also doubles as the first tab of the bottom navigation bar. This screen has two key states, the first being its intended display of the currently live and upcoming football matches. However, towards the end of a season, it is anticipated that there will be no upcoming or live matches to display, and in such a case, a simple message will replace the “loading…” text to convey this information.
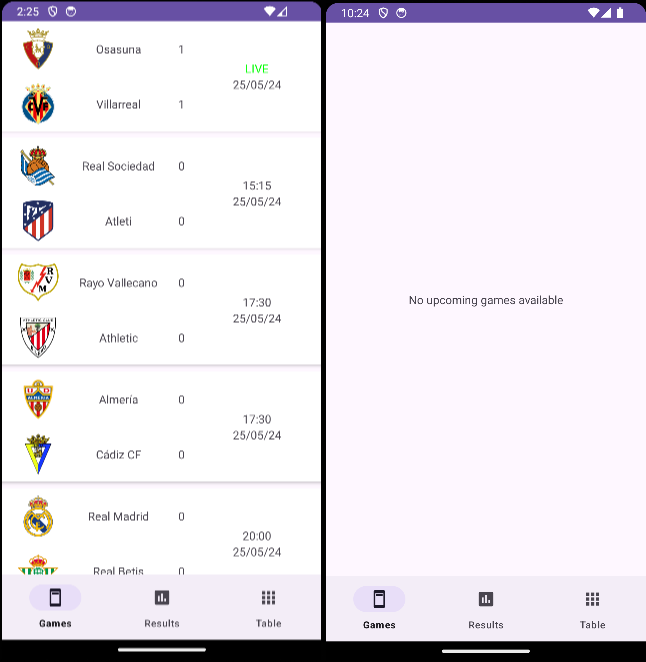
Progressing beyond the first screen is a relatively intuitive affair, simply requiring the press of one of the two other icons on the screen, which is clarified by marking the current screen that is being displayed. Using this navigation to move on to the “results” screen demonstrates a very similar presentation, but instead of showing current and upcoming matches, it instead shows matches that have been completed in their entirety along with the date they were played, making this tab a convenient way to view past fixtures spanning across an entire season.
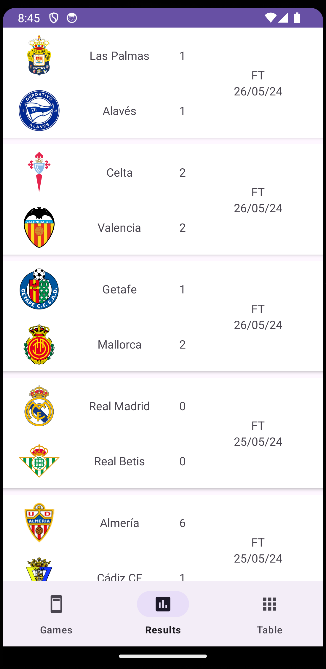
On both of the previous two screens, pressing on any fixture card summons a pop-up that contains more in-depth information about the selected fixture, such as the half-time score and host venue, which can then be just as easily dismissed by pressing on any part of the shaded area around the pop-up. This will also retain the current position of the base screen to prevent the frustration of losing scrolling progress after a quick check-in of one of the fixtures.
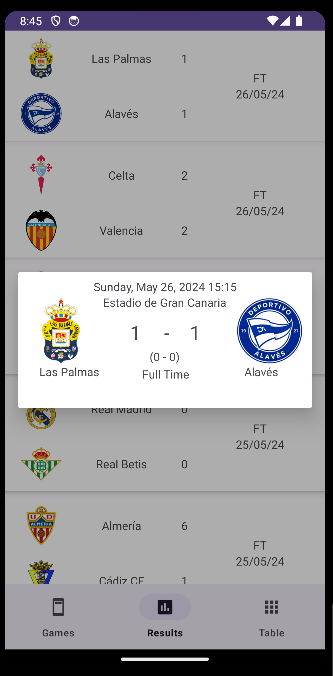
The final screen in the app is the league table. This screen is relatively simple, focusing on displaying a large amount of data, from team placement to goal statistics, in an easily digestible format. As a result, minimal user interaction is required, with only a scroll mechanic present to allow for intuitive table navigation.
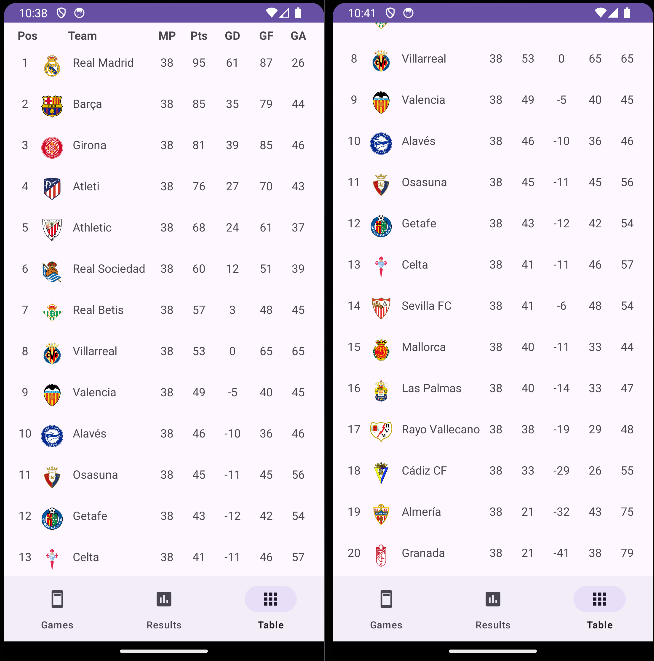